Introduction
PHPUnit is a unit testing framework for the PHP programming language. It is an instance of the xUnit architecture for unit testing frameworks that originated with SUnit and became popular with JUnit.
Unit Testing Flowchart:
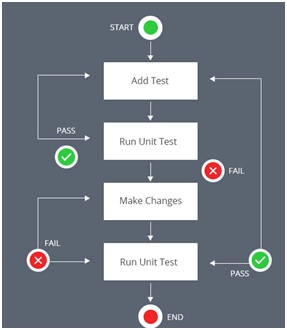
Other PHP E-commerce platforms
There are several other PHP E-commerce platforms WordPress, Magento, PrestaShop, OpenCart, Sylius, Aimeos and many more.
Click here to see our developed products where we have already used PHPUnit application testing
Basic Conventions to Write Unit Test Case
Following are some basic conventions and steps for writing tests with PHPUnit:
- Test File names should have a suffix Test. For example, if First.php needs to be tested,the name of the test file name will be FirstTest.php
- Similarly, If the class name is `MyFirstClass` than the test class name will be `MyFirstClassTest`.
- Add test as the prefix for method names. For example,if the method name is `getuser()`, then in test class, it will become `testgetuser()`. You can also use @test annotation in document block to declare it as a testing method.
- All testing methods are `public`
- MyFirstClassTest should be inherited from `PHPUnit\Framework\TestCase`
These are the major ground rules for the PHP unit testing framework. The essential configurations and settings are all set up. It is now time to write the first test case.
We will now start with a very simple test case.
Create a file FirstTest.php in UnitTestFiles/Test. Add the following code to it.
Code:
<?php
namespace UnitTestFiles\Test;
use PHPUnit\Framework\TestCase;
class FirstTest extends TestCase
{
public function testTrueAssetsToTrue()
{
$condition = true;
$this->assertTrue($condition);
}
}
?>
This is very basic test. In the first line, we mentioned the namespace where the test files are located.
Now in the UnitTestFiles/Test folder, create a new file called EmailTest.php and add the following test code:
Code:
<?php
declare(strict_types=1);
namespace UnitTestFiles\Test;
use PHPUnit\Framework\TestCase;
final class EmailTest extends TestCase
{
public function testCanBeCreatedFromValidEmailAddress(): void
{
$this->assertInstanceOf(
Email::class,
Email::fromString(‘user@example.com’)
);
}
public function testCannotBeCreatedFromInvalidEmailAddress(): void
{
$this->expectException(InvalidArgumentException::class);
Email::fromString(‘invalid’);
}
public function testCanBeUsedAsString(): void
{
$this->assertEquals(
‘user@example.com’,
Email::fromString(‘user@example.com’)
);
}
}
Here we have used assertInstanceOf() method which reports an error identified by $message if $actual is not an instance of $expected.