Introduction: Unraveling the Web Framework Maze
Embarking on a web development journey involves making critical decisions, and choosing the right framework is paramount. The maze of options includes Streamlit, Flask, and Django, each offering unique advantages. In this comprehensive guide, we delve into the nuances of these frameworks, providing a roadmap to navigate the complexities and make informed decisions for seamless and efficient development.
Section 1: Understanding Web Frameworks
Web frameworks are the backbone of web application development, streamlining the process and offering essential features. They provide a structured foundation for building and managing web applications, allowing developers to focus on implementing application-specific logic without reinventing the wheel for common web development tasks.
When evaluating different web frameworks, it’s important to consider factors such as scalability, flexibility, community support, and ease of use. These considerations play a crucial role in determining the most suitable framework for a particular project. Streamlit, Flask, and Django are three popular web frameworks, each with its own strengths and applications. Understanding the unique characteristics of these frameworks is key to making an informed decision when embarking on a web development project.
Section 2: Streamlit: A Beginner’s Guide
Streamlit, a relatively new addition to the web framework landscape, has gained traction for its focus on rapid prototyping and data visualization. Designed with simplicity and efficiency in mind, Streamlit empowers developers to create interactive web applications with minimal effort.
# Example Streamlit code for a simple data visualization app import streamlit as st import pandas as pd import matplotlib.pyplot as plt # Load sample data data = pd.DataFrame({ ‘Category’: [‘A’, ‘B’, ‘C’, ‘D’], ‘Values’: [4, 7, 1, 9] }) # Streamlit app st.title(‘Streamlit Data Visualization’) st.bar_chart(data.set_index(‘Category’)[‘Values’]) # Additional interactive elements selected_category = st.selectbox(‘Select a category:’, data[‘Category’]) st.write(f”You selected {selected_category}.”) |
One of Streamlit’s standout features is its ability to seamlessly integrate with popular data science libraries such as Pandas, Matplotlib, and Plotly. This integration enables developers to effortlessly generate visualizations and interactive interfaces for data analysis and model deployment. Additionally, Streamlit’s auto-reloading capability ensures that any changes to the code are immediately reflected in the application, saving valuable development time and enhancing the prototyping process.
Despite its emphasis on rapid development, Streamlit is not limited to small-scale projects. It can be used to build sophisticated web applications, particularly those focused on data exploration and visualization. Whether you’re a data enthusiast looking to share insights or a developer seeking a streamlined approach to web app creation, Streamlit presents a compelling option for bringing data-centric ideas to life.
Section 3: Flask: Harnessing the Power of Flexibility
Flask is renowned for its minimalist and extensible nature, offering developers the freedom to mold their applications according to specific requirements.
# Example Flask code for a minimal web application
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html', message='Hello, Flask!')
if __name__ == '__main__':
app.run(debug=True)
As a micro-framework, Flask provides the essential components for web development without imposing rigid structures or dependencies. This level of flexibility makes Flask an attractive choice for building lightweight applications and APIs, as well as for integrating with other technologies to create custom solutions.
With its modular design and extensive ecosystem of extensions, Flask empowers developers to tailor their applications with only the necessary components, avoiding unnecessary bloat and complexity. This minimalist approach fosters a high degree of customization, allowing developers to craft precise solutions that align with project objectives. Furthermore, Flask’s support for various databases and its compatibility with popular front-end frameworks make it a versatile tool for diverse web development scenarios.
In addition to its flexibility, Flask boasts a supportive community and a wealth of resources, including extensive documentation and tutorials. This accessibility contributes to the framework’s appeal, particularly for developers seeking a balance between simplicity and extensibility. Whether you’re building a small-scale web application or a RESTful API, Flask’s adaptability and resourcefulness make it a formidable contender in the realm of web development frameworks.
Section 4: Django: The Robust Framework for Scalable Applications
Django stands out as a full-featured web framework, renowned for its batteries-included approach and comprehensive set of built-in tools.
# Example Django code for a simple blog application
# models.py
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
pub_date = models.DateTimeField('date published')
# views.py
from django.shortcuts import render
from .models import Post
def index(request):
latest_posts = Post.objects.order_by('-pub_date')[:5]
context = {'latest_posts': latest_posts}
return render(request, 'blog/index.html', context)
# templates/blog/index.html
<!-- Loop through and display latest blog posts -->
{% for post in latest_posts %}
<h2>{{ post.title }}</h2>
<p>{{ post.content }}</p>
<p>Published on: {{ post.pub_date }}</p>
{% endfor %}
One of Django’s defining attributes is its emphasis on convention over configuration, promoting a standardized approach to web application development. This convention-driven nature simplifies the setup and configuration process, enabling developers to focus on application logic and business requirements. Furthermore, Django’s adherence to best practices, such as secure-by-default settings and built-in protection against common web vulnerabilities, contributes to the framework’s appeal for building secure and stable applications.
Django’s suitability for large-scale projects is further underscored by its support for database abstraction, internationalization, and asynchronous task execution. These features make it well-equipped to handle the complexities of enterprise-level applications and content-heavy websites. Additionally, Django’s thriving community and extensive documentation provide developers with a wealth of resources for honing their skills and overcoming development challenges.
Section 5: Comparing Streamlit, Flask, and Django
When weighing the characteristics of Streamlit, Flask, and Django, it’s essential to consider their respective strengths and limitations within the context of different project requirements.
Streamlit excels in rapid prototyping and data visualization, making it an excellent choice for showcasing data-driven insights and building interactive demos. Its simplicity and integration with data science libraries position it as a valuable tool for data-focused projects and quick application development.
Flask shines in its flexibility and minimalistic approach. It is well-suited for creating lightweight web applications and RESTful APIs, offering developers the freedom to tailor their applications with precision. Flask’s modular design and extensive ecosystem of extensions cater to a wide range of use cases, making it an adaptable choice for custom web solutions and integrations.
Django, with its batteries-included philosophy and comprehensive feature set, is best suited for building large-scale and complex web applications. Its emphasis on convention over configuration, combined with robust security features and built-in tools, makes it an ideal choice for enterprise-level projects and content-heavy websites. Django’s scalability, maintainability, and adherence to best practices position it as a framework of choice for projects requiring a high level of structure and standardization.
Looking for a Streamlit app development services? Enquire now!
Build Your Streamlit apps Now
We are here to customize your immediate needs and launch your product on time within the defined budget.
Please share your requirement and get ready to see how AKRATECH can build your MVP in no time.
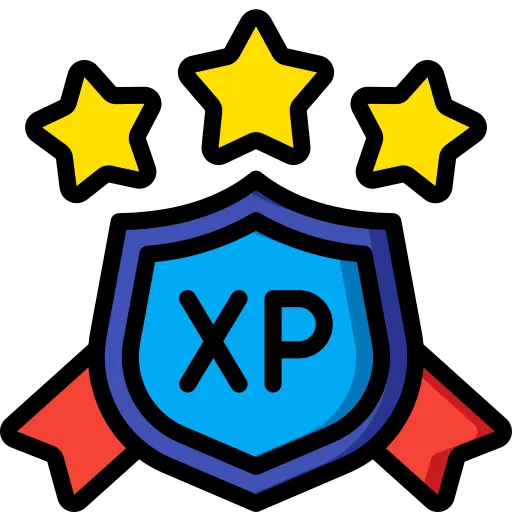
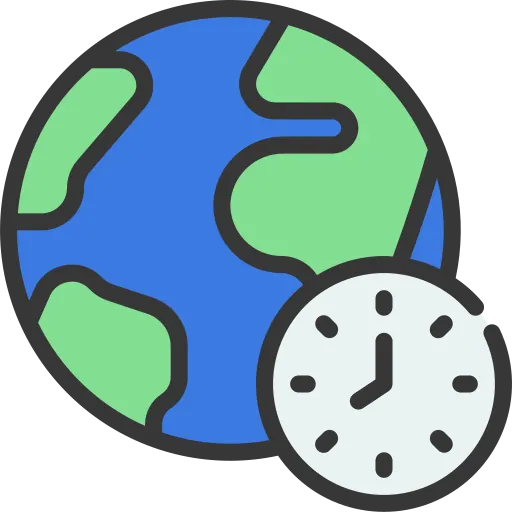
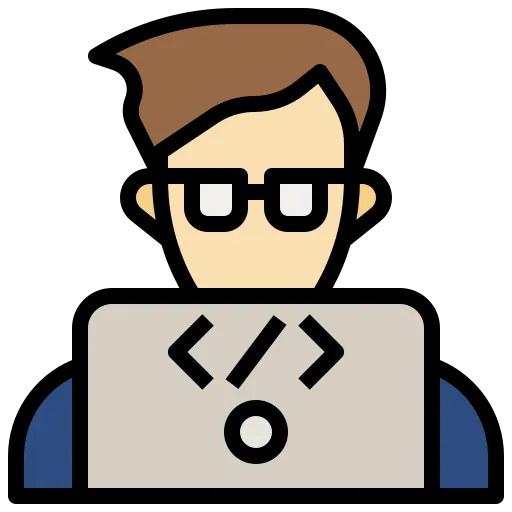
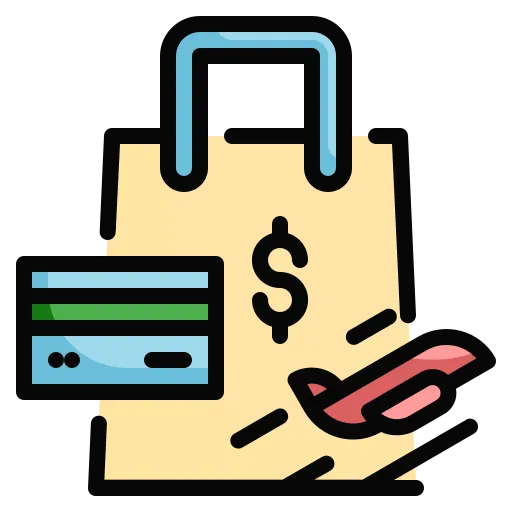
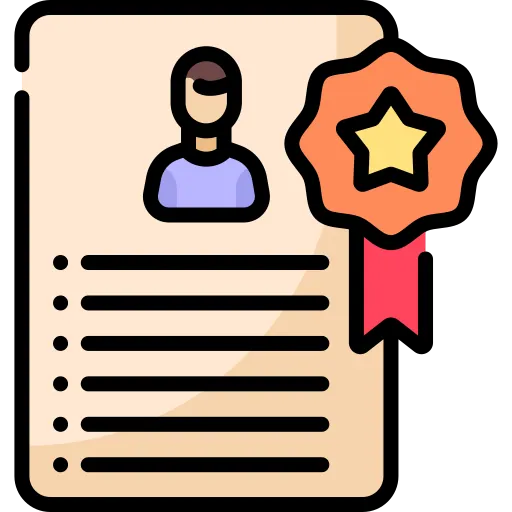
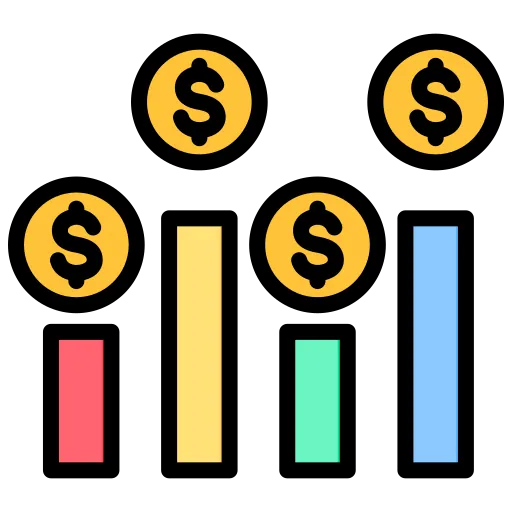
Section 6: Streamlit vs. Flask vs. Django: Performance and Scalability
When evaluating the performance and scalability of Streamlit, Flask, and Django, it’s essential to consider the specific requirements and constraints of the intended projects.
Streamlit, designed for rapid prototyping and data visualization, excels in scenarios where quick iterations and interactive interfaces are crucial. Its lightweight nature and focus on data-driven applications make it well-suited for projects with a strong emphasis on visualizing and exploring data insights.
# Example: Streamlit code for a performance-centric data visualization app
import streamlit as st
import numpy as np
# Generate synthetic data
data = np.random.randn(1000, 2)
# Streamlit app for scatter plot
st.title('Streamlit Performance Demo')
st.scatter_chart(data)
Flask, known for its flexibility and extensibility, offers respectable performance and scalability, particularly for small to medium-sized applications and RESTful APIs. Its minimalist design and modular architecture allow for efficient resource utilization and tailored solutions, making it a suitable choice for projects with specific performance requirements and custom integrations.
# Example: Flask code for a performant RESTful API
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
data = {'message': 'Data from Flask API'}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
Django, with its comprehensive feature set and emphasis on best practices, is engineered for scalability and performance in the context of large-scale applications and high-traffic websites. Its built-in tools, support for database abstraction, and asynchronous task execution contribute to its robust performance capabilities, making it an optimal choice for projects demanding high throughput, complex workflows, and extensive user interactions.
# Example: Django code for a scalable and performant web application
# views.py
from django.shortcuts import render
from .models import Post
def index(request):
latest_posts = Post.objects.order_by('-pub_date')[:5]
context = {'latest_posts': latest_posts}
return render(request, 'blog/index.html', context)
Section 7: Streamlit vs. Flask vs. Django: Community and Support
The strength of a framework’s community and the availability of support resources are instrumental in ensuring a smooth development experience and overcoming challenges.
Streamlit, as a relatively newer addition to the web framework landscape, has been rapidly gaining traction within the data science and machine learning communities. Its growing community and active user base contribute to the availability of resources, tutorials, and community-driven extensions, fostering a supportive environment for developers seeking to leverage its capabilities.
Flask benefits from a well-established community and a wealth of extensions, documentation, and tutorials. Its popularity among developers and its extensive ecosystem of third-party libraries and tools underscore the framework’s robust support network. The Flask community actively contributes to the framework’s evolution, ensuring a steady stream of updates, bug fixes, and best practices for developers to leverage.
Django, with its long-standing presence in the web development landscape, boasts a thriving community and an abundance of resources. The framework’s extensive documentation, official support channels, and a diverse range of third-party packages and integrations reflect its strong community backing. Additionally, Django’s community-driven initiatives, such as conferences, meetups, and forums, provide developers with ample opportunities to engage, learn, and collaborate with peers.
Section 8: Streamlit vs. Flask vs. Django: Learning Curve and Ease of Use
The learning curve and ease of use of a web framework are pivotal considerations for developers, particularly those seeking to expedite the development process and minimize complexities.
Streamlit, designed with simplicity in mind, offers an intuitive and beginner-friendly environment for creating data-driven web applications. Its declarative syntax, seamless integration with data science libraries, and auto-reloading capabilities contribute to a streamlined development experience, making it accessible to developers with varying levels of expertise.
# Example: Streamlit code for a beginner-friendly web app
import streamlit as st
# Streamlit app with a simple user interface
st.title('Streamlit Beginner App')
user_name = st.text_input('Enter your name:')
st.write(f'Hello, {user_name}!')
Flask, known for its minimalist design and flexibility, strikes a balance between ease of use and customization. Its straightforward API and extensive documentation enable developers to quickly grasp its core concepts and begin building applications with minimal friction. The modular nature of Flask allows developers to incrementally expand their knowledge and skills as they explore its ecosystem of extensions and integrations.
# Example: Flask code for a user-friendly web application
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route('/', methods=['GET', 'POST'])
def home():
if request.method == 'POST':
user_name = request.form['user_name']
return render_template('home.html', user_name=user_name)
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
Django, while renowned for its comprehensive feature set and conventions, may entail a steeper learning curve for developers new to the framework. However, Django’s emphasis on best practices, its standardized approach to web application development, and its extensive documentation contribute to a structured learning path for developers aiming to harness its capabilities.
Example: Django code for a structured and easy-to-understand web app
# views.py
from django.shortcuts import render
def home(request):
return render(request, 'home.html', {'user_name': 'Guest'})
# templates/home.html
<!-- Display a welcome message -->
<h1>Hello, {{ user_name }}!</h1>
Once familiar with Django’s conventions and patterns, developers can leverage its powerful features to build scalable and maintainable applications with confidence.
Conclusion: Your Roadmap to Efficient Web Development
This comprehensive guide has equipped you with the knowledge needed to make an informed decision when selecting a web framework for your next development venture. Each framework has its strengths and specializations, and by understanding their nuances, you can take confident steps towards achieving your web development goals. Say goodbye to decision paralysis and embrace the opportunities offered by Streamlit, Flask, and Django as you embark on your journey of seamless and efficient web development.