Introduction
Python is a popular programming language known for its simplicity and versatility. However, when it comes to building high-performance APIs with real-time data needs, Python might not be the first choice. But what if I told you that Python can be as fast as Node.js for API development? In this blog post, we’ll explore how you can achieve comparable speed to Node.js by using FastAPI and Asyncpg to create a blazing-fast and asynchronous API. We’ll also discuss the advantages of this setup and its use cases, like building admin dashboards or analytic pages. And finally, we’ll learn how to deploy the application using Docker.
Chapter 2: Getting Started
Before we dive into building a high-performance API, let’s set up our development environment.
Setting Up Your Development Environment:
To start building high-performance APIs with FastAPI and Asyncpg, you’ll need to ensure that your development environment is ready. Follow these steps:
- Install Python: If you haven’t already, you need to install Python. You can download it from the official Python website: Python Downloads.
- Choose a Code Editor: You’ll also need a code editor or integrated development environment (IDE) to write and run your Python code. Popular choices include Visual Studio Code, PyCharm, or Jupyter Notebook.
Installing FastAPI and Asyncpg:
With your development environment ready, you can install the necessary libraries for building high-performance APIs. Open your terminal or command prompt and run the following commands:
pip install fastapi
pip install uvicorn[standard]
pip install asyncpg
These commands will install:
- FastAPI, which is a high-performance web framework for building APIs.
- Uvicorn, an ASGI server for FastAPI.
- Asyncpg, a PostgreSQL database interface library optimized for asynchronous programming.
Creating Your First FastAPI Application:
Now that you have FastAPI and Asyncpg installed, it’s time to create your very first FastAPI application. Create a new Python file (e.g., app.py) and add the following code:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def read_root():
return {"message": "Hello, World"}
This code sets up a basic FastAPI application with a single endpoint that responds with a “Hello, World” message when you access the root URL.
To run your FastAPI application, use Uvicorn by running the following command in your terminal:
uvicorn app:app --host 0.0.0.0 --port 8000 --reload
After running this command, you can visit http://localhost:8000 in your web browser, and you should see the “Hello, World” message.
In the next chapter, we’ll explore the advantages of using FastAPI and Asyncpg for building high-performance APIs.
Chapter 3: Advantages of Using FastAPI and Asyncpg
In this chapter, we’ll discuss the key advantages of using FastAPI and Asyncpg for building high-performance APIs.
Asynchronous Programming:
FastAPI is built on top of Starlette and Pydantic, leveraging asynchronous programming to handle concurrent requests efficiently. Asynchronous code allows your application to perform non-blocking I/O operations, making it highly responsive and capable of handling multiple requests concurrently.
Automatic API Documentation:
FastAPI provides automatic interactive documentation through Swagger UI and ReDoc. This means you don’t have to manually document your APIs, as FastAPI generates documentation based on your code, including request and response models, validation rules, and endpoints.
Data Validation and Serialization:
FastAPI makes it easy to validate and serialize data. You can use Pydantic models to define the structure of your request and response data, and FastAPI will handle validation and serialization for you. This not only ensures data consistency but also helps prevent common API-related bugs.
Performance and Speed:
FastAPI is designed for high performance. It compiles your code to a highly efficient ASGI application, and its asynchronous nature allows it to handle a large number of concurrent requests. When paired with a performant database interface like Asyncpg, you can achieve impressive response times, making Python as fast as Node.js for many use cases.
In the next chapter, we’ll dive into building a high-performance API with FastAPI and Asyncpg, including asynchronous database access and real-time data handling.
Chapter 4: Building a High-Performance API
In this chapter, we’ll focus on the practical aspects of building a high-performance API using FastAPI and Asyncpg.
Handling Routes and Endpoints:
FastAPI makes it easy to define routes and endpoints. Routes are defined using decorators like @app.get(“/”) or @app.post(“/items/”). These decorators specify the HTTP method and URL path for each endpoint. For example:
@app.get("/items/{item_id}")
async def read_item(item_id: int):
return {"item_id": item_id}
This code defines a GET endpoint that expects an item_id parameter and returns a JSON response.
Asynchronous Database Access with Asyncpg:
To fetch data from a PostgreSQL database asynchronously, you can use Asyncpg. First, install it as mentioned in Chapter 2. Then, you can use it to connect to your database and perform queries asynchronously. For example:
import asyncpg
async def get_data_from_database():
conn = await asyncpg.connect(user='user', password='password', database='mydb', host='127.0.0.1')
result = await conn.fetch('SELECT * FROM mytable')
await conn.close()
return result
This code connects to a PostgreSQL database, fetches data, and closes the connection, all in an asynchronous manner.
Handling Real-time Data Requests:
FastAPI and Asyncpg excel at handling real-time data requests. You can create endpoints that return real-time data, such as live analytics or sensor data, with minimal latency. The asynchronous nature of FastAPI and Asyncpg ensures that your application can handle multiple concurrent data requests efficiently.
In the next chapter, we’ll explore the use cases for this high-performance API, including admin dashboards and analytic pages.
Looking to hire a Python developer?
Get top-tier talent to bring your projects to life with precision and efficiency!
Ready to find the perfect fit for your team? Contact us today and start building your dream solutions!
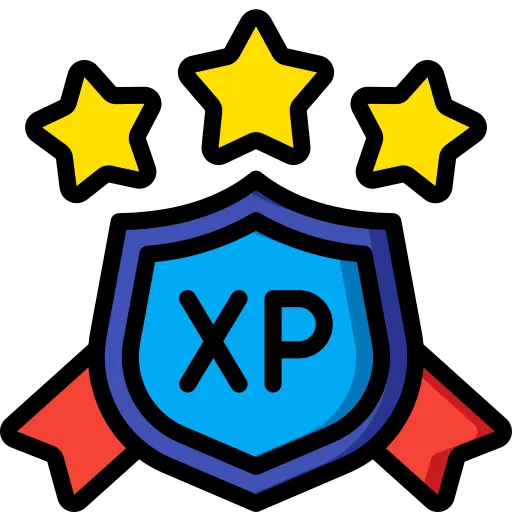
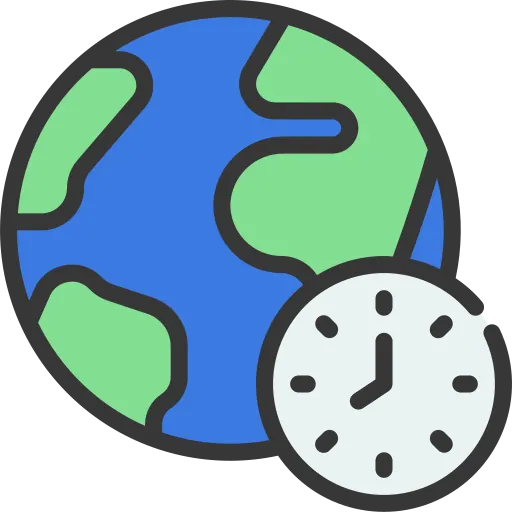
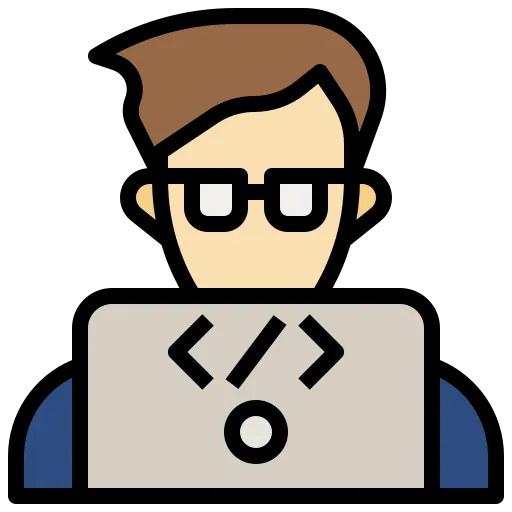
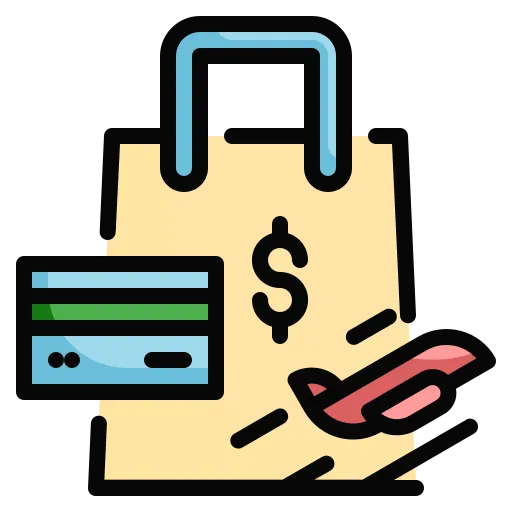
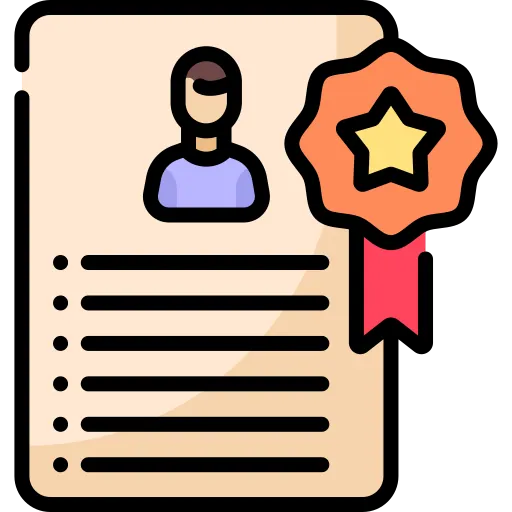
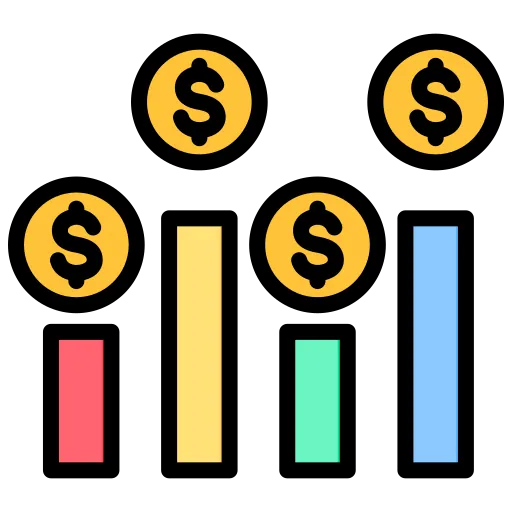
Chapter 5: Use Cases
FastAPI and Asyncpg are versatile tools that can be used in various use cases. Here are a few examples of where this high-performance setup can shine:
Admin Dashboards:
Admin dashboards often require real-time access to application data, user statistics, or system health metrics. FastAPI’s speed and asynchronous capabilities make it an excellent choice for building responsive admin dashboards that update in real-time.
Analytic Pages:
Analytic pages need to fetch and display large amounts of data quickly. FastAPI’s efficient data validation and serialization, combined with Asyncpg’s database performance, ensure that you can build fast and reliable analytic pages.
Real-time Data Dashboards:
Whether you’re monitoring stock prices, weather data, or social media trends, real-time data dashboards demand speed and responsiveness. FastAPI and Asyncpg are ideal for building dashboards that deliver data as it’s generated or updated.
IoT Applications:
Internet of Things (IoT) applications often involve handling real-time sensor data. FastAPI’s asynchronous features enable you to process and serve IoT data efficiently, making it a perfect fit for IoT projects.
In the next chapter, we’ll learn how to deploy your high-performance API using Docker, ensuring scalability and ease of management.
Chapter 6: Deployment with Docker
Deploying your FastAPI application with Docker ensures a consistent and manageable environment. Docker containers package your application and its dependencies, making it easy to scale and deploy across different environments.
Introduction to Docker:
Docker is a platform for developing, shipping and running applications in containers. Containers are lightweight, portable, and include everything needed to run a piece of software, including the code, runtime, libraries, and system tools.
Creating a Docker Image for Your FastAPI Application:
To create a Docker image for your FastAPI application, you’ll need to create a Dockerfile. This file specifies how your application should be packaged into a container. Here’s a basic example:
# Use an official Python runtime as a parent image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Copy the requirements file into the container
COPY requirements.txt requirements.txt
# Install any needed packages specified in requirements.txt
RUN pip install -r requirements.txt
# Copy the rest of your application code into the container
COPY . .
# Define the command to run your application
CMD ["uvicorn", "app:app", "--host", "0.0.0.0", "--port", "8000"]
docker build -t my-fastapi-app
This command builds a Docker image named “my-fastapi-app” from the current directory.
Deploying Your Application in Containers:
Once you have a Docker image, you can run it as a container. Here’s an example command:
docker run -d -p 8000:8000 my-fastapi-app
This command runs the “my-fastapi-app” container in detached mode, mapping port 8000 from the container to port 8000 on your host machine.
Scaling Your Application with Docker Compose:
If you need to run multiple containers or services for your application, you can use Docker Compose. Docker Compose allows you to define and run multi-container Docker applications. You can specify your FastAPI application, database, caching, and other services in a docker-compose.yml file and start them together.
In the next chapter, we’ll cover testing, monitoring, and debugging your FastAPI application to ensure its reliability and performance.
Chapter 7: Testing and Monitoring
Testing and monitoring are critical aspects of building a high-performance API with FastAPI and Asyncpg. In this chapter, we’ll discuss how to write unit tests for your FastAPI endpoints, monitor and optimize your API’s performance, and handle debugging.
Writing Unit Tests for FastAPI Endpoints:
FastAPI makes it easy to write unit tests for your API endpoints using Python’s built-in unittest framework or popular testing libraries like PyTest. You can simulate HTTP requests and validate the responses to ensure your endpoints work as expected. Comprehensive testing helps catch bugs and ensures your API remains reliable.
Monitoring and Optimizing API Performance:
Monitoring your API’s performance is essential to identify bottlenecks and areas for improvement. Tools like Prometheus and Grafana can help you collect and visualize performance metrics. Profiling your code with tools like cProfile can uncover performance issues that need attention. Optimizing code, database queries, and caching strategies can significantly enhance your API’s speed and responsiveness.
Debugging with FastAPI:
FastAPI provides helpful debugging features. You can enable debugging mode during development to receive detailed error information in responses, making it easier to identify and fix issues.
Chapter 8: Conclusion
In this blog post, we’ve explored how FastAPI and Asyncpg can transform Python into a high-performance API development platform. We’ve covered the advantages of using FastAPI, such as its asynchronous capabilities, automatic documentation, data validation, and speed.
We’ve also delved into building a high-performance API with FastAPI and Asyncpg, handling routes, asynchronous database access, and real-time data requests. We’ve discussed use cases for this setup, including admin dashboards, analytic pages, real-time data dashboards, and IoT applications.
We’ve shown how to deploy your FastAPI application using Docker, ensuring scalability and ease of management. We’ve also highlighted the importance of testing, monitoring, and debugging to maintain a reliable and efficient API.
With the power of FastAPI and Asyncpg, Python can compete with Node.js in the world of high-performance APIs. The future looks bright for Python developers aiming to build blazing-fast, real-time applications.