What Are Streamlit, Flask and Django?
Streamlit, Flask and Django are three frameworks used for building web applications. Streamlit is a toolbox for creating interactive, performant user interfaces, while Flask is a microframework that enables developers to create simple yet powerful applications quickly. Django is a comprehensive framework with built-in features like authentication, object-relational mappers and others that enable developers to build complex applications quickly and efficiently.
How does Streamlit work?
Streamlit is an open-source framework for building interactive, data-driven applications in Python. It provides a simple, easy-to-use interface for creating beautiful, interactive web apps with just a few lines of Python code. It uses a simple, syntax-based approach to building interactive web apps. You can create an app by writing a Python script that uses the Streamlit library to create UI elements like sliders, dropdown menus, and text boxes.
Getting started with Streamlit is quick and easy. To create a new Streamlit app, you first need to install the Streamlit library using pip:
pip install streamlit
Once you’ve installed Streamlit, you can start a new app by running the following command in your terminal:
streamlit run app.py
This will start a local web server that will host your Streamlit app. You can access the app in your web browser by navigating to http://localhost:8501.
Streamlit app examples
Machine Learning Model Deployment: Streamlit makes it easy to deploy machine learning models and provide an interactive interface for users to make predictions. You can create a simple Streamlit app that loads a trained machine learning model and allows users to input data and receive predictions in real-time.
Data Exploration and Visualization: Streamlit’s API makes it easy to create interactive data visualizations. For example, you can build an app that allows users to explore and visualize a large dataset, with options to filter and aggregate data, and to view visualizations in real-time.
Geospatial Data Visualization: Streamlit can be used to visualize geospatial data, such as maps and satellite imagery. You can create an app that allows users to view geospatial data and interact with it, for example by changing the map view, selecting specific regions, or overlaying additional data.
Financial Dashboard: Streamlit can be used to create financial dashboards that allow users to monitor and analyze financial data in real-time. For example, you can build an app that retrieves stock market data and displays it in various visualizations, including line charts, bar charts, and candlestick charts.
These are just a few examples of the types of Streamlit apps you can build. With its simple and intuitive API, Streamlit makes it easy to build and deploy a wide range of interactive data apps.
Advantages of Using Streamlit to Build Web Applications
Streamlit is one of the fastest growing frameworks for building web applications. It offers many advantages over traditional web development frameworks such as Flask and Django, including its interactive user interfaces that provide a more intuitive experience, as well as its built-in features which enable developers to rapidly develop complex applications in less time. Streamlit also boasts lightning-quick page loads that keep users engaged and on the page, further reducing development time and costs.
flask web application architecture
The architecture of a Flask web application is typically comprised of the following components:
Models: Models represent the data structures in your application, and they are usually defined using an Object-Relational Mapping (ORM) library such as SQLAlchemy.
Views: Views handle incoming requests and return responses to the client. In Flask, views are defined as functions that take a request and return a response.
Templates: Templates define the structure and layout of the HTML pages in your application. Flask uses the Jinja2 template engine, which allows you to include dynamic content in your templates, such as variables and control structures.
Forms: Forms allow users to input data into your application. In Flask, forms can be created using the WTForms library, which provides a convenient way to define form fields, validate user input, and render forms in HTML.
Routes: Routes define the URLs of your application and map them to specific views. In Flask, you define routes using the @app.route decorator.
Static Files: Static files, such as CSS and JavaScript files, are used to enhance the visual appearance and functionality of your application. In Flask, you can define a static directory in your application where static files can be stored and served to the client.
Database: The database stores the data used by your application. Flask can be used with a variety of databases, including SQLite, PostgreSQL, and MySQL.
These components work together to create a complete web application. When a user makes a request to your application, Flask uses the routing information to determine which view should handle the request. The view retrieves any necessary data from the database and renders the appropriate template, which is then returned to the user as an HTML response.
Looking for Python development services?
Harness the power of Python to create robust, scalable solutions tailored to your needs.
Ready to bring your vision to life? Contact us today and let our experts transform your ideas into reality!
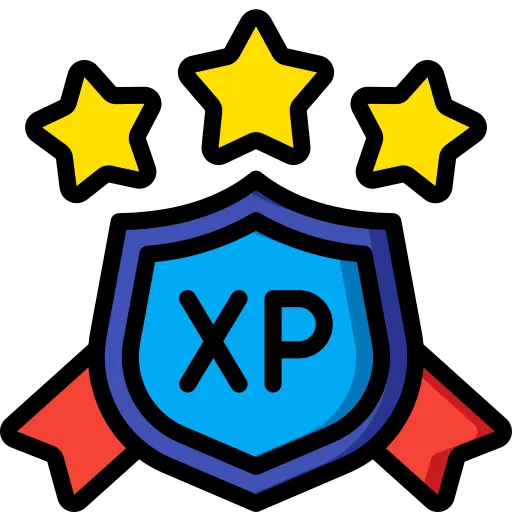
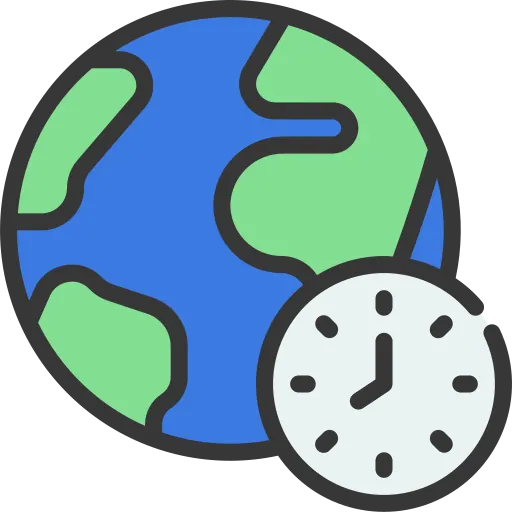
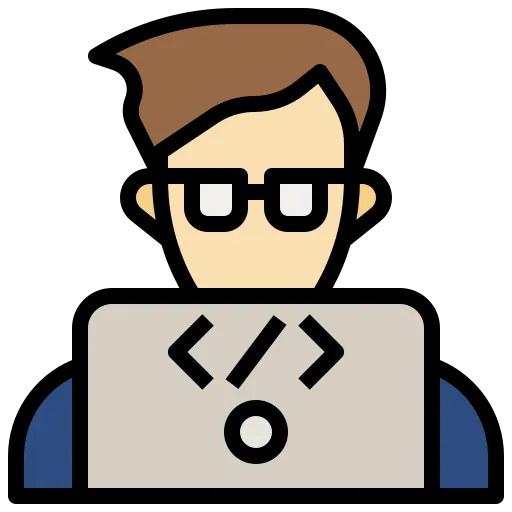
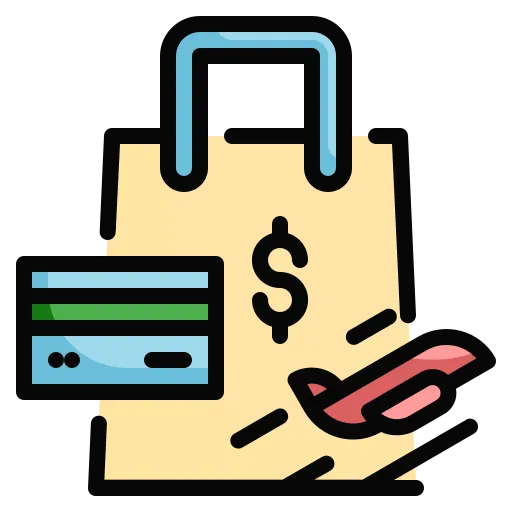
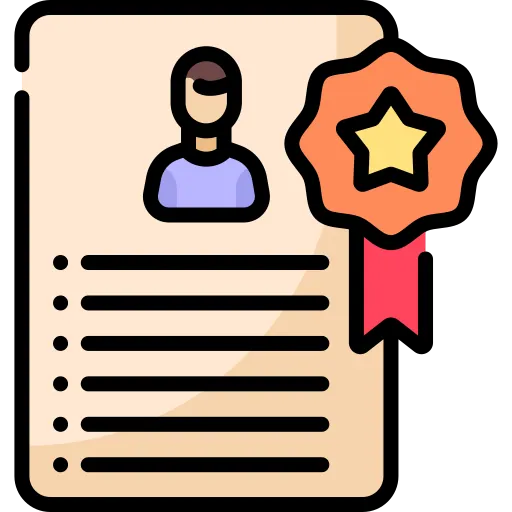
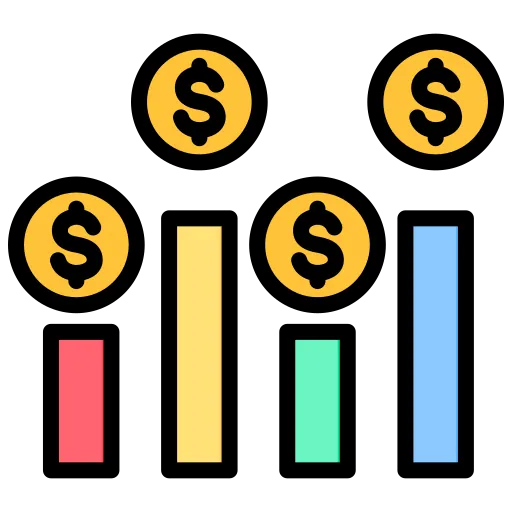
High-level workflow for building a Streamlit app:
- Install Streamlit: If you haven’t already done so, install Streamlit by running the following command in your terminal or command prompt –
pip install streamlit
- Create a new Python file: Create a new Python file and import the Streamlit library at the beginning of the file: –
import streamlit as st
- Define the layout: Use Streamlit’s layout functions, such as st.title(), st.header(), st.subheader(), and st.write(), to define the structure of your app and display any text or data.
- Create interactive widgets: Use Streamlit’s interactive widgets, such as sliders, dropdowns, checkboxes, and radio buttons, to allow users to interact with your app and change its behavior. You can use functions like st.slider(), st.selectbox(), st.checkbox(), and st.radio() to create these widgets.
- Define app logic: Use Python code to define the logic of your app. For example, you might load data from a file or database, perform some data analysis or machine learning, or generate visualizations. You can use any Python library in your Streamlit app.
- Run the app: To run your Streamlit app, open a terminal or command prompt, navigate to the directory containing your Python file, and run the following command: –
streamlit run your_file_name.py
This will start a local server and open your app in a web browser. You can then interact with your app and see how it behaves. - Share your app: If you want to share your app with others, you can deploy it to a cloud-based platform like Heroku or AWS. Streamlit also offers its own hosting platform called Streamlit Sharing, which allows you to deploy and share your apps for free. To deploy your app, follow the deployment instructions provided by your hosting platform.
how flask app works
A Flask app works by handling HTTP requests and returning HTTP responses. When a user makes a request to a Flask app, the following steps occur:
- The request is received by the Flask app and passed to the appropriate view function, based on the URL of the request.
- The view function retrieves any necessary data from the database, processes the data as needed, and then renders a template.
- The template is used to generate an HTML response, which is then returned to the user’s browser.
- The user’s browser renders the HTML and displays the page to the user.
The flow of a Flask app is controlled by a combination of routes and view functions. Routes define the URLs of your application and map them to specific view functions. View functions handle incoming requests and return responses to the client.
When a user requests a URL, Flask uses the routing information to determine which view function should handle the request. The view function then retrieves any necessary data from the database and generates a response, which is returned to the user’s browser.
In this way, Flask provides a flexible and extensible framework for building web applications. With its simple and intuitive API, Flask makes it easy to build and deploy a wide range of web applications, from simple single-page apps to complex multi-page applications.
Advantages of Using Flask to Build Web Applications
Flask is a powerful web framework that allows developers to build dynamic applications quickly and easily. It’s highly extensible and integrates well with other tools, making it a great choice for building complex applications. With its built-in templating system and library, developers can quickly create robust applications. Plus, Flask’s code remains readable even when dealing with larger projects or heavier coding logic, which saves time for developers and keeps codebases maintainable.
Flask workflow
- Install Flask: If you haven’t already done so, install Flask by running the following command in your terminal or command prompt:
- Create a new Python file: Create a new Python file and import the Flask library at the beginning of the file:
from flask import Flask - Create an instance of the Flask class: Instantiate the Flask class to create a new Flask application. You can pass the name of the application to the Flask constructor. For example:
app = Flask(name)
- Define routes: Use the @app.route() decorator to define routes for your application. A route is a URL that a user can visit to interact with your application. You can define a route and the function that should handle that route. For example:
@app.route('/)
def index():
return 'Hello, World!'
This code defines a route that maps to the root URL of your application (/). When a user visits that URL, the index() function is called, which returns the string “Hello, World!”. - Run the app: To run your Flask app, you can add the following code at the end of your Python file:
if__name__=='__main__':
app.run()
This code starts a local development server on your computer and allows you to test your app in a web browser. You can then navigate to the URL of your application to see it in action. - Expand the app: Once you have a basic Flask app running, you can expand it by adding new routes, HTML templates, database connections, and more. Flask has a rich ecosystem of plugins and extensions that can help you add functionality to your app. You can also use any Python library in your Flask app.
- Deploy the app: If you want to share your Flask app with others, you can deploy it to a cloud-based platform like Heroku or AWS. To deploy your app, you typically need to create a production-ready configuration file, such as gunicorn or uWSGI, and configure it to run your Flask application. You will also need to set up a production database, if your app requires one.
django web application development
Django is a high-level Python web framework that enables rapid development of secure and maintainable web applications. The architecture of a Django web application typically consists of the following components:
Models: Models define the structure of the data in your application and are typically defined using Django’s Object-Relational Mapping (ORM) layer.
Views: Views handle incoming requests and return responses to the client. In Django, views are defined as Python functions or classes that take a request and return a response.
Templates: Templates define the structure and layout of the HTML pages in your application. Django uses the Django Template Language (DTL), which allows you to include dynamic content in your templates, such as variables and control structures.
Forms: Forms allow users to input data into your application. In Django, forms can be created using the Django Forms framework, which provides a convenient way to define form fields, validate user input, and render forms in HTML.
URLs: URLs define the address of your application and map them to specific views. In Django, you define URLs using regular expressions in a URL configuration file.
Middleware: Middleware is a component that sits between the request and response and can modify the request or response. In Django, you can use middleware to perform tasks such as authentication, caching, and compression.
Admin Site: Django provides an automatically generated administrative interface for your application, which allows you to manage the data stored in your models.
These components work together to create a complete web application. When a user makes a request to your application, Django uses the URL configuration to determine which view should handle the request. The view retrieves any necessary data from the database and renders the appropriate template, which is then returned to the user as an HTML response.
Django provides a rich set of tools and features for building web applications, including an ORM, an admin site, and built-in support for user authentication, URL routing, and more. This makes it a popular choice for web developers who want to build high-quality, scalable, and maintainable web applications quickly and efficiently.
difference between app and project django
In Django, a project refers to a collection of settings for an instance of a Django application. A project can contain one or more apps, which are individual components of a project that can be reused across multiple projects.
Here are some key differences between a Django project and app:
Scope: A project defines the overall configuration for a Django application, including the settings for the database, middleware, installed apps, and more. An app, on the other hand, is focused on a specific functionality and can be used as a standalone component in multiple projects.
Reusability: An app is designed to be reusable, meaning that it can be used in multiple projects without modification. A project, on the other hand, is specific to a particular instance of a Django application.
Structure: A project has a specific directory structure, including a settings.py file that contains the configuration for the project, while an app has a different structure that includes its own models, views, and templates.
Maintenance: Because an app is designed to be reusable, it is typically easier to maintain and upgrade. A project, on the other hand, may require more maintenance as it is tied to a specific instance of a Django application.
In summary, a Django project defines the overall configuration and settings for an instance of a Django application, while an app defines a specific component of that application that can be reused across multiple projects. The use of apps in Django allows developers to create modular and reusable components, which can make it easier to maintain and upgrade large-scale web applications.
Advantages of Using Django to Build Web Applications
Django is a favorite among developers thanks to its scalability, flexibility, and full-featured libraries. With its model-view-template (MVT) architectural pattern, applications built with Django stay organized and maintainable even as they become larger or more complex. Additionally, developers can leverage lots of ready-made components like user authentication and payments integration. Furthermore, the object relational mapper (ORM) allows Django apps to work with a variety of databases for storing data in different formats– so it’s possible to future-proof an application without significant overhead.
Django workflow
- Install Django: If you haven’t already done so, install Django by running the following command in your terminal or command prompt:
pip install Django
- Create a new Django project: Create a new Django project by running the following command in your terminal or command prompt:
django-admin startproject project_name
This will create a new directory called project_name with a basic Django project structure. - Create a new Django app: Create a new Django app within your project by running the following command in your terminal or command prompt:
python manage.py startapp app_name
This will create a new directory called app_name with a basic Django app structure. - Define models: Define the models for your app using Django’s built-in Object-Relational Mapping (ORM). Models represent the data that your application will store in a database. You define models as Python classes that inherit from django.db.models.Model. For example:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=200)
pub_date = models.DateTimeField('date published') - Create database tables: Once you have defined your models, you can create the database tables that correspond to those models by running the following command in your terminal or command prompt:
python manage.py migrate
- Define views: Define the views for your app. Views are Python functions that handle HTTP requests and return HTTP responses. You can define a view for a specific URL pattern using Django’s URL routing system. For example:
from django.http import HttpResponse
def index(request):return HttpResponse("Hello, world. You're at the polls index.")
- Create templates: Define HTML templates that your views can use to generate dynamic content. Django uses a templating system that allows you to write templates that include variables, loops, conditionals, and other dynamic content.
- Define URL patterns: Define the URL patterns for your app. URLs are mapped to views using Django’s URL routing system. You can define URL patterns using regular expressions and include named groups that can be passed as arguments to your views. For example:
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
] - Run the app: To run your Django app, navigate to the top-level directory of your project and run the following command in your terminal or command prompt:
python manage.py runserver
This will start a local development server and allow you to test your app in a web browser. You can then navigate to the URL of your application to see it in action. - Expand the app: Once you have a basic Django app running, you can expand it by adding new models, views, templates, and URL patterns. Django has a rich ecosystem of plugins and extensions that can help you add functionality to your app.
- Deploy the app: If you want to share your Django app with others,
Comparative Analysis Between Streamlit, Flask and Django
Each of these frameworks offer their own unique advantages and it can be hard to tell which one works best for a given project. Streamlit is an ideal choice if you want to quickly create lightweight ML-driven applications, while Flask is better suited for smaller projects where custom code will overwrite the built-in functionality. Django, on the other hand, offers more features but may not be as efficient when working with small data sets or simple tasks. The right choice really depends on how much control you need over each feature and your budget.
Streamlit, Flask, and Django are all web frameworks for building web applications using Python. However, they differ in their design philosophies and target use cases. Here are some key differences between these frameworks:
Design philosophy:
Streamlit: Streamlit is designed to be a simple and easy-to-use framework for building data-driven applications, particularly data science applications. Streamlit provides a simple API for building interactive web applications without requiring much web development experience.
Flask: Flask is designed to be a lightweight and flexible web framework that can be used for a variety of use cases, including building simple web applications, REST APIs, and microservices. Flask provides a minimalist core and extensible plug-ins that can be used to add additional functionality.
Django: Django is designed to be a full-featured and powerful web framework that provides a lot of built-in functionality for building complex web applications, including user authentication, database management, and content management. Django is often used for building large-scale applications that require a lot of built-in features and security.
Ease of use:
Streamlit: Streamlit is very easy to use and requires minimal setup. You can quickly build and deploy data-driven applications without requiring a lot of web development experience.
Flask: Flask is also easy to use, but requires a bit more setup than Streamlit. Flask provides a minimal core that can be extended with plug-ins, which can make it a bit more complex to get started with.
Django: Django can be more challenging to set up and requires a more complex development environment. However, once you have set up Django, it provides a lot of built-in features and tools that can make development easier and faster.
Customization:
When customizing Streamlit, Flask, and Django for web development, each framework offers distinct levels of flexibility and built-in features tailored to various project requirements and developer expertise. Streamlit, known for its simplicity and focus on data-driven applications, provides a straightforward approach to building interfaces but with limited customization compared to the highly flexible Flask and feature-rich Django frameworks. Understanding the nuances of each can empower developers to choose the most suitable tool for their specific needs and customization preferences.
Streamlit: Streamlit provides a limited set of UI components and is designed to be used primarily for data-driven applications. It is not as customizable as Flask or Django.
Flask: Flask provides a minimal core that can be extended with plug-ins to add additional functionality. This makes Flask very customizable and flexible, but also requires more development expertise.
Django: Django provides a lot of built-in functionality and tools for building complex web applications. It is highly customizable and provides a lot of options for extending its built-in features.
Scalability:
Streamlit: Streamlit is designed primarily for building small to medium-sized applications and may not scale well to very large applications.
Flask: Flask can be used for building applications of any size, but may require more work to scale up to very large applications.
Django: Django is designed to be used for building large-scale applications and provides a lot of built-in features for managing application scalability.
In summary, Streamlit, Flask, and Django are all great web frameworks for building web applications using Python, but they differ in their design philosophies and target use cases. Streamlit is best for building small to medium-sized data-driven applications, Flask is best for building flexible and customizable applications, and Django is best for building large-scale applications with a lot of built-in features.